Lecture Overview
- HTML Forms
- Input Elements
- Response Forms
- The
<form>
element is used to retrieve data from a user.
- A
form
can contain different types of input elements.
<body>
<form> </form>
</body>
- Input elements are child elements of the
form
element.
- Input elements are displayed depending on the
type
attribute-value.
- There are 4 types of Input
- Text Input Field
- Text Input Submit Button
- Radio Button Input
- Checkbox Input Button
Text Input Field
<input type = "text">
- Specifies a one-line input field for text input
<form>
First name:
<br>
<input type="text" id="firstname">
<br>
Last name:
<br>
<input type="text" id="lastname">
</form>
Text Input Submit Button
<input type="submit">
- Defines a button for submitting form data to a form-handler
- Form-handler is usually a server page with a script for processing input data
- The form-handler is specified in the
form
’s action
attribute
<form id="myform" action="JavaScript:myFunctionName()">
First name:<br>
<input type="text" id="firstname" ><br>
Last name:<br>
<input type="text" id="lastname" ><br><br>
<input type="submit" value="Submit">
</form>
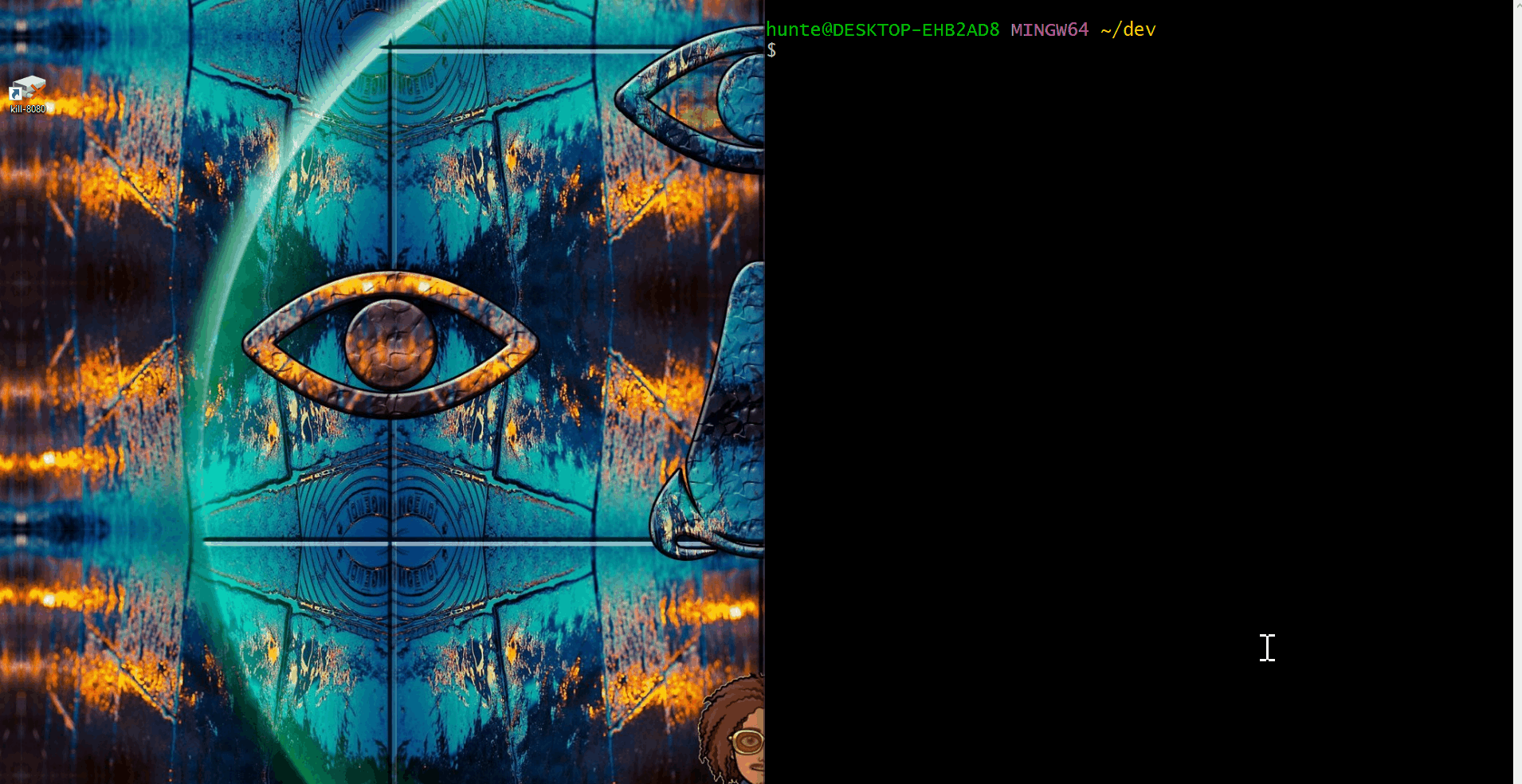
- Buttons written in
HTML
can perform actions defined in JavaScript
.
- Ensuring that the
onClick
event return false
will prevent the DOM
from refreshing after the function is called.
- The code snippet written below is JavaScript written within the same file between the
<script>
tags.
- It should be noted that JavaScript does not have to be written explicitly within the same file.
- JavaScript can be written in external files, then added into HTML using an expression like the example below
<script type="text/javascript" src="./app.js"><script>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" cont`ent="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Name Echoer</title>
<script type="text/javascript">
function evaluateUserInput(){
let userInputFirstName = document.getElementById("first_name").value;
let userInputLastName = document.getElementById("last_name").value;
let outputDisplay = "Your name is: " + userInputFirstName + " " + userInputLastName;
document.getElementById("output").innerHTML = outputDisplay;
return false;
}
</script>
</head>
<body>
<form id="myform">
First name:<br><input id="first_name" type="text"><br>
Last name:<br><input id="last_name" type="text"><br>
<button id="submit_button" onClick="return evaluateUserInput();">Submit</button>
</form>
<p id="output">placeholder text until the `submit_button` is clicked</p>
</body>
</html>
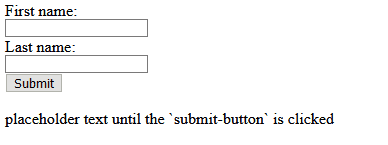
- Below is another example of a responsive form performs basic arithmetic
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Calculator</title>
<script type="text/javascript">
function evaluateUserInput() {
let refreshDomAfterTriggeringEvent = false;
let firstValue = document.getElementById("first_value").value;
let secondValue = document.getElementById("second_value").value;
let operation = document.getElementById("operation").value;
let expression = firstValue + operation + secondValue;
let output = eval(expression);
document.getElementById("output").innerHTML = output;
return refreshDomAfterTriggeringEvent
}
</script>
</head>
<body>
<form id="myform">
First name:<br/><input id="first_name" type="text"/><br/>
Last name:<br/><input id="last_name" type="text"/><br/>
<button id="submit_button" onClick="return evaluateUserInput();">Submit</button>
</form>
<p id="output">placeholder text until the `submit_button` is clicked</p>
</body>
</html>