CSS and Styling
Lecture Overview
- What is CSS?
- How to insert CSS
- How CSS is applied
- Styling with Fonts, Color, and Size
What is CSS and why do we use it?
- CSS stands for Cascading Style Sheets.
- CSS describes how HTML elements are to be displayed on screen, paper, or in other media
- CSS can control the layout of multiple web pages at once
CSS Syntax
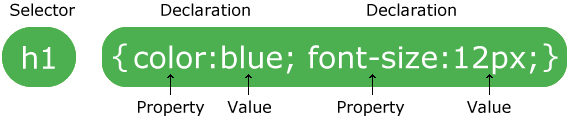
- CSS selectors points to the HTML elements you want to style
- A CSS declaration always ends with a semicolon, and declaration blocks are surrounded by curly braces
- A property indicates which stylistic features you want to change. Ex: Font, width, background color
- A value indicated how you want to change the stylistic feature
CSS Selectors
- CSS selectors are used to select HTML elements based on their element attribute and/or element position.
Element Selectors
- The element selector selects elements based on the element name
- The code below selects all
<p>
elements on a page and makes them center aligned and red
p {
text-align: center;
color: yellow;
}
Id Selectors
- The id selector uses the id attribute of an HTML element to select a specific element
- The id of an element should be unique within a page
- id selector is used to select a single HTML element
- Use a hash
#
followed by the id of the element
#headshot {
background: green;
border: 1px solid red;
}
Class Selectors
- The class selector selects elements with a specific class attribute
- Use a period
.
character, followed by the name of the class
- Can appear multiple times within a page, unlike id selectors which only appear once
.projects {
text-align: center;
}
Grouping Selectors
- If multiple elements have the same style, they can be concatenated as a single selector delimited by commas
- This helps to minimize code
h1, h2, p {
text-align: center;
color: red;
}
Descendant Selectors
- Descendant selectors (or descendant combinator) allow you to combine two or more selectors so you can be more specific
- Elements matched by the second selector are selected if they have an ancestor element matching the first selector
- This relationship is created with a space between selectors
ul li {
margin: 10px;
}
How to insert CSS
- There are three ways to insert CSS
- External Style Sheet
- Internal Style Sheet
- Inline style
External Style Sheet
- external stylesheets stylize the page by referencing css written in a separate file with a
.css
extension
- include a reference to the external style sheet file inside the
<link>
element.
- The
<link>
element is placed in the <head>
section by convention
- Changes to an external stylesheet file will affect the style of every page referencing the file.
<head>
<link rel="stylesheet" type="text/css" href="mystyle.css">
</head>
Internal Style Sheet
- internal stylesheets stylize the page by referencing css written in the same file in the
<head>
tag.
- Changes to an internal stylesheet will only affect the style of the page embedding the css.
<head>
<style>
body { background-color: blue; }
p { color: white; }
</style>
</head>
Inline Style
- The inline style is added as an HTML attribute.
<h1 style="font-size: 48px;">My headline</h1>
How CSS is applied
Cascading
- Styles are read from top to bottom in the stylesheet
- The lowest style “wins”
p {
color: red;
font-weight: bold;
font-size: 18px;
}
p {
color: blue;
}
<p>I’m blue, 18px, and bold.</p>
Inheritance
- Some styles are passed from parent to children
p {
color: red;
font-weight: bold;
font-size: 18px;
}
span {
font-style: italic;
}
<p>I’m red, 18px, and bold.
<span>I am those and also italic.</span>
</p>
CSS Specificity
- If two CSS selectors apply to the same element, the one with higher specificity wins
- There are 4 categories which define the specificity level of a selector
- Each category has a certain number of points
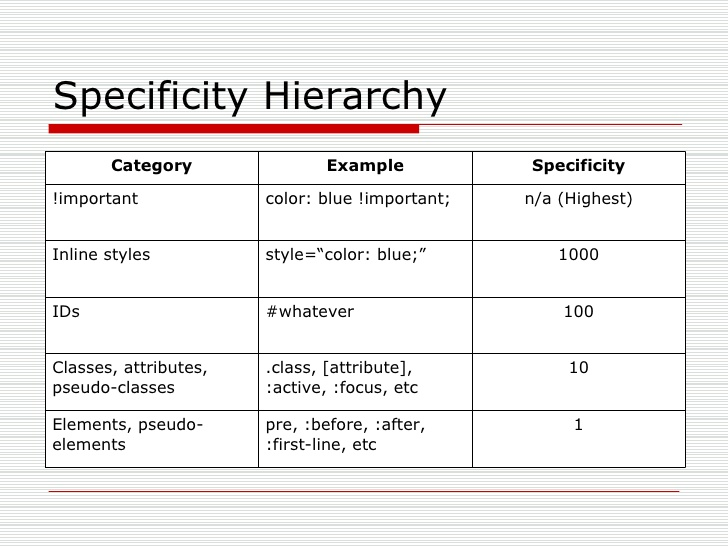
Inline styles
- An inline style is directly attached to the element that is styled
- Has a value of 1000 points
<h1 style="color: #ffffff;">
IDs
- A unique identifier for the page elements using
#
- Has a value of 100 points
#headshot {
background: green;
border: 1px solid red;
}
Classes, attributes, and pseudo-classes
- This category includes
.classes
, [attributes]
and pseudo-classes such as :hover
, :focus
etc.
.project{
font-family: Tahoma;
font color: #4F0E42;
}
a:hover{
color: purple;
}
Elements and pseudo-elements
- This includes element names and pseudo-elements, such as
h1
, div
, :before
and :after
.
- Has a value of 1 point
h2{
font-family: Comic Sans, sans-serif;
font color: #DFAEB4;
}
Specificity Chart
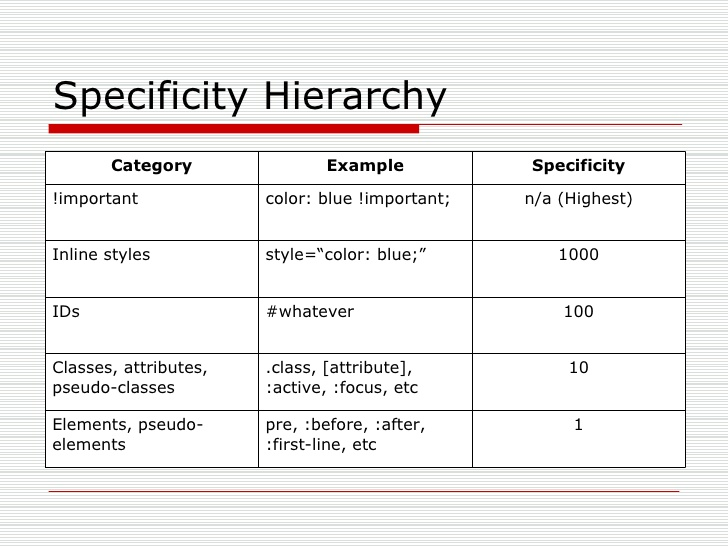
CSS Styling with Fonts, Size, and Colors
- Various fonts, sizes, and colors can be used through CSS to style your HTML web page
Font-family
font-family
property assigns a font to the specified element(s)
font-family
property can hold several font names as a “fallback” system.
- If the browser does not support the first font, it tries the next font.
- Property should hold several font names as a fallback system if the browser does not support the first font.
body {
/* A font family name and a generic family name */
font-family: "Arial", sans-serif;
font-family: "Times New Roman", serif;
/* A generic family name */
font-family: serif;
}
Difference Between Serif and Sans-serif
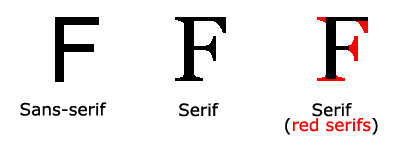
Font-Size
font-size
sets the size of the font
12px
- 14px
is recommended for the body text accessibility
p {
/* <length> values */
font-size: 12px;
/* <percentage> values */
font-size: 80%;
}
Font
- The
font
property is shorthand for font-style
, font-variant
, font-weight
, font-size
, and font-family
- Shorthand properties allow you to set multiple values with one declaration
p {
font: italic bold 12px "Arial", sans-serif;
}
Color
- The
color
property is used to set the color of the text
- Colors in CSS can be specified using
- a color name -
red
- a HEX value -
#ff0000
- an RGB or RGBA value -
rgb(255)
p {color: red;}
Background-Color
- The
background-color
property changes the color of the Background
p {
background-color: black;
background-color: #000000;
background-color: rgba(0,0,0);
}
Text-Align
- The
text-align
property is used to set the horizontal alignment of a text
- Text can be
left
, right
, centered
, justify
alignment
- When the
text-align
property is set to justify
, each line is stretched so that every line has equal width and the left and right margins are straight.
- Similar to magazines and newspapers
h1 {
text-align: center;
}
p {
text-align: left;
}
.right {
text-align: right;
}
div {
text-align: justify;
}
A list of all the CSS properties
- https://css-tricks.com/almanac/properties/